Unlocking the Power of MERN Stack: From Beginner to Expert
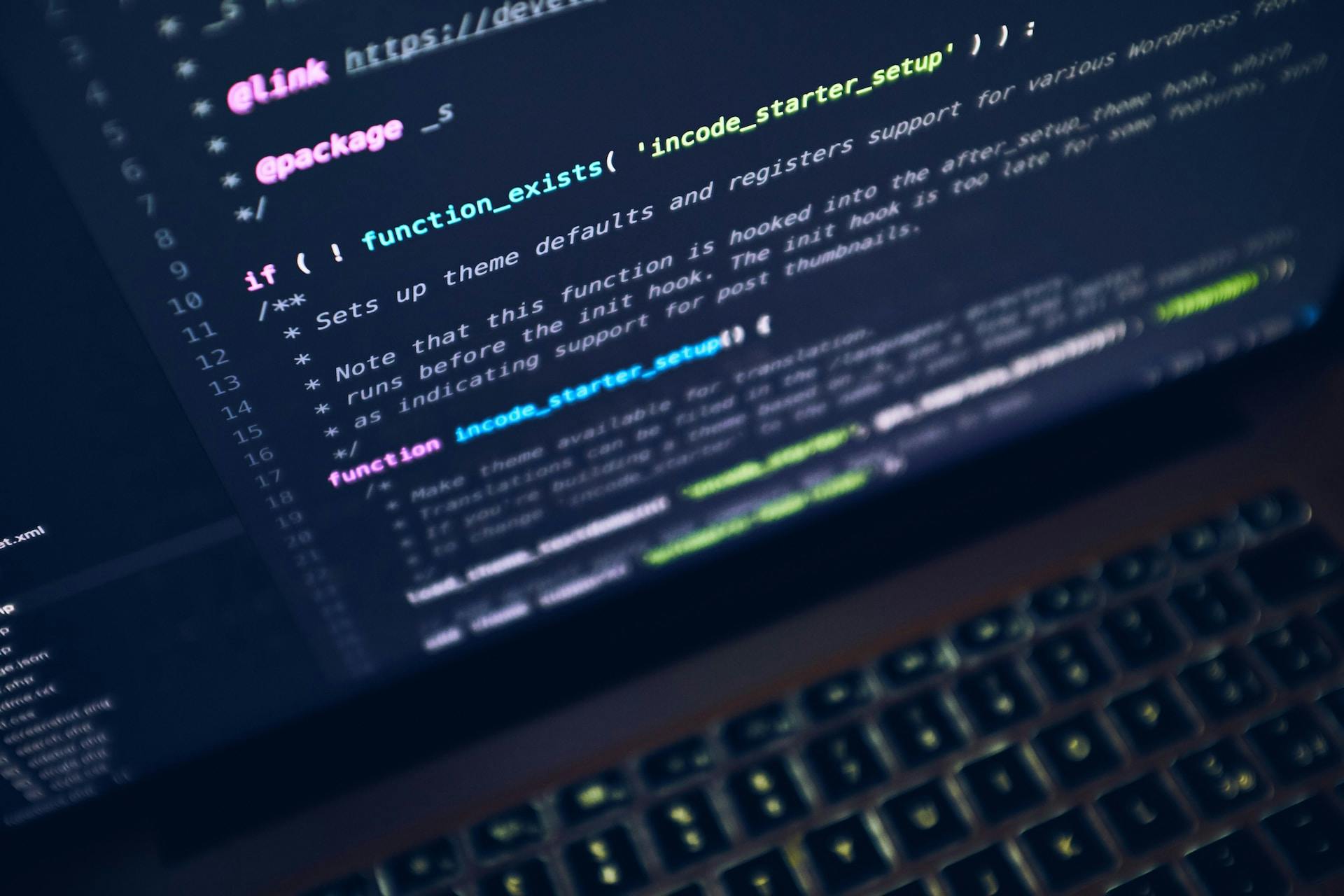
Table Of Content
- Introduction:
- Chapter 1: Introduction to MERN Stack
- Chapter 2: Setting Up Your Development Environment
- Chapter 3: Understanding MongoDB
- Chapter 4: Exploring Express.js
- Chapter 5: Mastering React.js
- Chapter 6: Navigating Node.js
- Running tests with Mocha
- Chapter 7: Building Your First MERN Application
- Chapter 8: Advanced React Components and State Management
- Chapter 9: RESTful API Development with Express.js
- Chapter 10: Real-Time Communication with WebSockets
- Chapter 11: Securing Your MERN Stack Applications
- Chapter 12: Optimizing Performance in MERN Stack Applications
- Chapter 13: Deploying and Scaling MERN Stack Applications
- Chapter 14: Summary and Further Learning
- Chapter 15: Conclusion
Introduction:
Welcome to "Unlocking the Power of MERN Stack: From Beginner to Expert." In this comprehensive guide, we embark on a journey through the MERN Stack, a powerful combination of technologies comprising MongoDB, Express.js, React.js, and Node.js, revolutionizing the world of web development. Whether you're a novice programmer or an experienced developer looking to expand your skill set, this book is your ultimate companion to mastering every aspect of building dynamic, modern web applications.
With over 500 pages of in-depth content, "Unlocking the Power of MERN Stack" provides a step-by-step roadmap to navigate through the complexities of each component, guiding you from the foundational concepts to advanced techniques. Through practical examples, hands-on exercises, and real-world projects, you'll gain the expertise needed to create robust, scalable, and efficient web applications using the MERN Stack.
Chapter 1: Introduction to MERN Stack
The MERN Stack is a full-stack JavaScript framework that enables developers to build dynamic web applications entirely in JavaScript. Comprising MongoDB, Express.js, React.js, and Node.js, this stack offers a seamless development experience from server to client.
Understanding the Components:
- MongoDB: A NoSQL database that stores data in flexible, JSON-like documents, making it ideal for handling large volumes of data and scaling horizontally.
- Express.js: A minimalist web application framework for Node.js that simplifies the process of building web servers and APIs, providing robust features for routing, middleware, and HTTP utilities.
- React.js: A JavaScript library for building user interfaces, known for its component-based architecture, declarative syntax, and efficient rendering, enabling developers to create interactive UIs with ease.
- Node.js: A JavaScript runtime environment that executes server-side code, allowing developers to build scalable and high-performance web applications using JavaScript on both the client and server sides.
Why Choose MERN Stack:
- Unified Language: With MERN Stack, developers can use JavaScript for both frontend and backend development, streamlining the development process and reducing context switching.
- Rich Ecosystem: Each component of the MERN Stack has a vibrant ecosystem of libraries, tools, and community support, empowering developers to leverage pre-built solutions and accelerate development.
- Flexibility and Scalability: MERN Stack offers flexibility in choosing components and scaling applications, allowing developers to adapt to changing requirements and handle increased traffic seamlessly.
Getting Started:
To begin your journey with MERN Stack, ensure you have Node.js and npm (Node Package Manager) installed on your system. Additionally, familiarity with JavaScript, HTML, and CSS is recommended for a smoother learning experience.
In the upcoming chapters, we'll dive deeper into each component of the MERN Stack, exploring their functionalities, best practices, and real-world applications. By the end of this book, you'll possess the knowledge and skills to embark on your MERN Stack projects with confidence and expertise.
Chapter 2: Setting Up Your Development Environment
Before delving into the intricacies of the MERN Stack, it's crucial to set up your development environment properly. A well-configured environment ensures smooth development workflow and facilitates collaboration with other developers. In this chapter, we'll walk through the steps to set up your development environment for MERN Stack development.
Choosing an Integrated Development Environment (IDE):
Selecting the right IDE can significantly impact your productivity and coding experience. While there are numerous options available, some popular choices among MERN Stack developers include:
- Visual Studio Code (VS Code): A lightweight and feature-rich code editor developed by Microsoft, equipped with powerful extensions for JavaScript, React, Node.js, and MongoDB development.
- Atom: A hackable text editor maintained by GitHub, offering customizable themes, packages, and a vibrant community.
- Sublime Text: A sophisticated text editor known for its speed, ease of use, and extensive plugin ecosystem, making it an excellent choice for MERN Stack development.
Regardless of the IDE you choose, ensure it supports JavaScript syntax highlighting, code completion, and integrated terminal for seamless development.
Installing Node.js and npm:
Node.js serves as the runtime environment for executing JavaScript code on the server side. To install Node.js, visit the official Node.js website and download the latest version compatible with your operating system. Node.js comes bundled with npm, the package manager for JavaScript, allowing you to install and manage dependencies for your MERN Stack projects effortlessly.
Once Node.js is installed, open your terminal or command prompt and verify the installation by running the following commands:
node -v
npm -v
These commands should display the installed versions of Node.js and npm, respectively.
Setting Up MongoDB:
MongoDB is a crucial component of the MERN Stack, serving as the database for storing and managing application data. To set up MongoDB locally, follow these steps:
- Download the MongoDB Community Server from the official MongoDB website and install it on your system.
- Create a data directory where MongoDB will store its data files.
- Start the MongoDB server by running the
mongod
command in your terminal.
Once MongoDB is up and running, you can interact with it using the MongoDB shell or a graphical user interface (GUI) tool like MongoDB Compass.
Configuring Express.js and React.js Projects:
To create and manage Express.js and React.js projects, you can use tools like Express Generator and Create React App, respectively. These tools scaffold out the project structure and provide a solid foundation for building MERN Stack applications.
- Express Generator: Install Express Generator globally using npm by running
npm install -g express-generator
. Then, create a new Express.js project usingexpress my-project-name
. - Create React App: Create a new React.js project by running
npx create-react-app my-app
in your terminal. This command sets up a new React.js project with all the necessary dependencies and configuration.
With your development environment set up and configured, you're now ready to embark on your journey of mastering the MERN Stack. In the subsequent chapters, we'll delve deeper into each component of the stack, exploring their functionalities, best practices, and real-world applications.
Chapter 3: Understanding MongoDB
MongoDB is a cornerstone of the MERN Stack, providing a flexible and scalable NoSQL database solution for modern web applications. In this chapter, we'll delve into the fundamental concepts of MongoDB, exploring its document-oriented data model, querying capabilities, and advanced features.
**Document-Oriented Data Model: **
Unlike traditional relational databases, MongoDB adopts a document-oriented data model, storing data in flexible, JSON-like documents known as BSON (Binary JSON). These documents can contain nested structures, arrays, and various data types, offering greater flexibility and schema-less design.
Consider the following example of a MongoDB document representing a user profile:
{
"_id": ObjectId("5fdaa890c8420b3b1c6a3e2a"),
"username": "john_doe",
"email": "[email protected]",
"age": 30,
"address": {
"city": "New York",
"country": "USA"
},
"interests": ["programming", "hiking", "photography"]
}
Each document in MongoDB is uniquely identified by a primary key called _id
, which ensures its uniqueness within the collection.
Querying Data in MongoDB:
MongoDB provides a powerful query language and rich set of operators for retrieving and manipulating data. Some commonly used query operators include:
- $eq: Matches values that are equal to a specified value.
- $gt: Matches values that are greater than a specified value.
- $in: Matches any of the values specified in an array.
- $regex: Performs a regular expression pattern match.
For example, to find all users with age greater than 25, you can use the following query:
db.users.find({ "age": { $gt: 25 } });
Indexes and Performance Optimization:
Indexes play a crucial role in optimizing query performance in MongoDB. By creating indexes on fields frequently used in queries, you can significantly improve query execution time and enhance overall database performance. MongoDB supports various types of indexes, including single-field indexes, compound indexes, and multi-key indexes.
To create an index on the username
field in the users
collection, you can use the following command:
db.users.createIndex({ "username": 1 });
Aggregation Framework:
MongoDB's Aggregation Framework provides powerful tools for performing data aggregation operations, such as grouping, sorting, and filtering, directly within the database. This enables developers to perform complex data transformations and analytics tasks efficiently.
For example, to calculate the average age of users in a collection, you can use the following aggregation pipeline:
db.users.aggregate([
{ $group: { _id: null, avgAge: { $avg: "$age" } } }
]);
Transactions and Atomicity:
MongoDB introduced multi-document transactions in version 4.0, allowing developers to perform atomic operations across multiple documents within a single transaction. This ensures data consistency and integrity, especially in scenarios involving multiple write operations.
In the next chapter, we'll explore Express.js, a minimalist web application framework for Node.js, and learn how to build robust backend APIs for MERN Stack applications.
Chapter 4: Exploring Express.js
Express.js is a minimalist web application framework for Node.js, designed to simplify the process of building robust and scalable web servers and APIs. In this chapter, we'll dive into the core concepts of Express.js, exploring routing, middleware, error handling, and more.
Setting Up an Express.js Application:
To create a new Express.js application, start by initializing a new Node.js project using npm:
npm init -y
Next, install Express.js as a dependency:
npm install express
With Express.js installed, you can create a new JavaScript file, such as app.js
, and require Express:
const express = require('express');
const app = express();
const port = 3000;
Routing in Express.js:
Express.js provides a simple and intuitive mechanism for defining routes and handling HTTP requests. Routes in Express.js are defined using HTTP methods (GET, POST, PUT, DELETE) and URL patterns.
// Define a route for handling GET requests to the homepage
app.get('/', (req, res) => {
res.send('Welcome to the homepage!');
});
Middleware in Express.js:
Middleware functions are functions that have access to the request object (req), the response object (res), and the next middleware function in the application’s request-response cycle. They can perform tasks such as logging, authentication, error handling, etc.
// Middleware function to log request details
app.use((req, res, next) => {
console.log(`${req.method} ${req.url}`);
next();
});
Error Handling in Express.js:
Express.js provides built-in error handling mechanisms to gracefully handle errors that occur during request processing. Error-handling middleware functions are defined with four arguments: (err, req, res, next)
.
// Error-handling middleware function
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something broke!');
});
Static File Serving:
Express.js allows you to serve static files, such as HTML, CSS, and client-side JavaScript, from a directory using the express.static
middleware.
// Serve static files from the 'public' directory
app.use(express.static('public'));
Express.js Middleware Ecosystem:
Express.js boasts a rich ecosystem of middleware modules that extend its functionality and provide additional features for various purposes. Some popular middleware modules include:
- Body-parser: Parses incoming request bodies in a middleware before your handlers, available under the req.body property.
- Helmet: Helps secure Express.js apps by setting various HTTP headers.
- Cors: Enables Cross-Origin Resource Sharing (CORS) in Express.js applications.
- Morgan: HTTP request logger middleware for Node.js, providing detailed request logging.
With a solid understanding of Express.js fundamentals, you're well-equipped to build robust backend APIs for your MERN Stack applications. In the next chapter, we'll dive into mastering React.js, the JavaScript library for building user interfaces, and explore its component-based architecture, state management, and more.
Chapter 5: Mastering React.js
React.js is a JavaScript library for building user interfaces, renowned for its component-based architecture, declarative syntax, and efficient rendering. In this chapter, we'll delve into the core concepts of React.js, exploring components, JSX, state management, and more.
Understanding Components:
Components are the building blocks of React.js applications, encapsulating reusable pieces of UI. React components can be either functional or class-based, and they can represent anything from simple buttons to complex UI elements.
// Functional component example
function Welcome(props) {
return <h1>Hello, {props.name}!</h1>;
}
// Class-based component example
class Welcome extends React.Component {
render() {
return <h1>Hello, {this.props.name}!</h1>;
}
}
JSX (JavaScript XML):
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within JavaScript, making it easier to describe the structure of UI components. JSX gets transpiled to regular JavaScript function calls by tools like Babel.
// JSX example
const element = <h1>Hello, world!</h1>;
State and Lifecycle:
State allows React components to manage their own data and update their UI in response to user actions or other events. Class-based components have a state object, while functional components can use the useState hook introduced in React 16.8.
// Class-based component with state
class Clock extends React.Component {
constructor(props) {
super(props);
this.state = { date: new Date() };
}
render() {
return <div>Current time: {this.state.date.toLocaleTimeString()}</div>;
}
}
Handling Events:
React.js provides a synthetic event system that normalizes events across different browsers. Event handlers in React are similar to traditional HTML event handlers but written in camelCase.
// Event handling example
function handleClick() {
console.log('Button clicked!');
}
<button onClick={handleClick}>Click me</button>
Conditional Rendering:
React allows you to conditionally render components or elements based on certain conditions using JavaScript expressions or ternary operators.
// Conditional rendering example
function Greeting(props) {
const isLoggedIn = props.isLoggedIn;
return isLoggedIn ? <UserGreeting /> : <GuestGreeting />;
}
Forms and Controlled Components:
React components can also manage form elements and their state using controlled components, where form data is controlled by React instead of the DOM.
// Controlled component example
class NameForm extends React.Component {
constructor(props) {
super(props);
this.state = { value: '' };
}
handleChange(event) {
this.setState({ value: event.target.value });
}
handleSubmit(event) {
alert('A name was submitted: ' + this.state.value);
event.preventDefault();
}
render() {
return (
<form onSubmit={this.handleSubmit}>
<input type="text" value={this.state.value} onChange={this.handleChange} />
<button type="submit">Submit</button>
</form>
);
}
}
With a solid understanding of React.js fundamentals, you're well-prepared to build dynamic and interactive user interfaces for your MERN Stack applications. In the next chapter, we'll navigate through Node.js, the JavaScript runtime environment, and explore its capabilities for building server-side applications and APIs.
Chapter 6: Navigating Node.js
Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine, allowing developers to run JavaScript code on the server side. In this chapter, we'll explore the core concepts of Node.js, including asynchronous programming, modules, file system operations, and building RESTful APIs.
Asynchronous Programming with Callbacks:
Node.js employs an event-driven, non-blocking I/O model, making it well-suited for handling concurrent operations efficiently. Asynchronous programming in Node.js is typically achieved using callbacks, allowing functions to execute asynchronously and call another function once the operation completes.
// Asynchronous file read operation using callbacks
const fs = require('fs');
fs.readFile('file.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
Modules and Module System:
Node.js uses a module system to organize code into reusable units called modules. Each module encapsulates related functionality and can expose variables, functions, or classes for use in other modules using the module.exports
object.
// Exporting a function from a module
module.exports = function greet(name) {
return `Hello, ${name}!`;
};
// Importing a function from a module
const greet = require('./greet');
console.log(greet('John'));
File System Operations:
Node.js provides built-in modules for interacting with the file system, enabling reading from and writing to files, creating directories, and performing other file-related operations.
// Writing data to a file
const fs = require('fs');
fs.writeFile('message.txt', 'Hello, world!', (err) => {
if (err) throw err;
console.log('File written successfully!');
});
Building RESTful APIs with Express.js:
Node.js, in conjunction with Express.js, enables developers to build powerful and scalable RESTful APIs for serving data to client applications. Express.js provides middleware functions for handling HTTP requests, routing, and other essential features.
// Example of defining routes in Express.js
const express = require('express');
const app = express();
app.get('/api/users', (req, res) => {
// Logic to fetch users from the database
res.json(users);
});
app.post('/api/users', (req, res) => {
// Logic to create a new user
res.status(201).json(newUser);
});
// Start the Express.js server
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
Debugging and Testing:
Node.js offers robust debugging and testing capabilities, allowing developers to debug their applications using built-in debugging tools or third-party libraries like node-inspect
. Additionally, testing frameworks like Mocha and Jest facilitate unit testing, integration testing, and end-to-end testing of Node.js applications.
# Running tests with Mocha
npm install mocha --save-dev
mocha tests/*.test.js
With Node.js as the backend powerhouse of your MERN Stack applications, you can seamlessly handle server-side logic, data manipulation, and API interactions. In the following chapters, we'll dive deeper into building full-stack applications with MERN Stack, leveraging the power of MongoDB, Express.js, React.js, and Node.js together.
Chapter 7: Building Your First MERN Application
Now that we've explored the core concepts of MongoDB, Express.js, React.js, and Node.js individually, it's time to bring them together to build your first MERN (MongoDB, Express.js, React.js, Node.js) application. In this chapter, we'll guide you through the process of setting up a basic MERN application, creating a simple CRUD (Create, Read, Update, Delete) interface, and deploying it to a server.
Setting Up the Project Structure:
Start by creating a new directory for your MERN project and navigating into it:
mkdir mern-project
cd mern-project
Next, initialize a new Node.js project and install the necessary dependencies:
npm init -y
npm install express mongoose react react-dom
Create subdirectories for the backend and frontend components of your application:
mkdir backend frontend
Setting Up the Backend (Express.js with MongoDB):
Navigate into the backend
directory and create a new file named server.js
. This file will serve as the entry point for your Express.js backend.
// backend/server.js
const express = require('express');
const mongoose = require('mongoose');
const app = express();
const PORT = process.env.PORT || 5000;
// MongoDB connection
mongoose.connect('mongodb://localhost:27017/mern-app', {
useNewUrlParser: true,
useUnifiedTopology: true
}).then(() => {
console.log('Connected to MongoDB');
}).catch((err) => {
console.error('Error connecting to MongoDB:', err);
});
// Define routes
app.get('/', (req, res) => {
res.send('Hello from the backend!');
});
// Start the server
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Setting Up the Frontend (React.js):
Navigate into the frontend
directory and set up a new React.js project using Create React App:
npx create-react-app client
This command will scaffold a new React.js project inside the frontend/client
directory.
Creating a Simple CRUD Interface:
In the React.js frontend, you can create components to perform CRUD operations, such as displaying a list of items, adding new items, updating existing items, and deleting items. You can use Axios or Fetch API to make HTTP requests to the Express.js backend to handle these operations.
// frontend/client/src/components/TodoList.js
import React, { useState, useEffect } from 'react';
import axios from 'axios';
const TodoList = () => {
const [todos, setTodos] = useState([]);
useEffect(() => {
axios.get('/api/todos')
.then(response => {
setTodos(response.data);
})
.catch(error => {
console.error('Error fetching todos:', error);
});
}, []);
return (
<div>
<h2>Todo List</h2>
<ul>
{todos.map(todo => (
<li key={todo._id}>{todo.title}</li>
))}
</ul>
</div>
);
};
export default TodoList;
Deploying Your MERN Application:
Once you've developed and tested your MERN application locally, you can deploy it to a server using platforms like Heroku, AWS, or DigitalOcean. Make sure to configure the necessary environment variables, set up a production build for the frontend, and ensure that your MongoDB database is accessible from the deployed server.
With your MERN application deployed, you've successfully built and launched your first full-stack web application using MongoDB, Express.js, React.js, and Node.js. In the subsequent chapters, we'll explore advanced topics and techniques to enhance your MERN Stack development skills further.
Chapter 8: Advanced React Components and State Management
In this chapter, we'll delve deeper into React.js by exploring advanced concepts such as higher-order components, hooks, and state management libraries. These techniques will enable you to build more modular, efficient, and scalable React applications.
Higher-Order Components (HOCs):
Higher-order components are functions that accept a component as input and return a new component with enhanced functionality. They allow for code reuse and logic abstraction across multiple components.
// Example of a higher-order component
const withAuthentication = (Component) => {
class WithAuthentication extends React.Component {
render() {
if (this.props.isAuthenticated) {
return <Component {...this.props} />;
} else {
return <Login />;
}
}
}
return WithAuthentication;
};
// Usage: Wrap a component with the higher-order component
const ProfilePage = withAuthentication(Profile);
React Hooks:
Hooks are functions that enable functional components to use state and other React features without writing a class. They provide a more concise and readable way to manage state, side effects, and context within functional components.
// Example of useState hook for managing state
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
State Management with Context API:
The Context API provides a way to pass data through the component tree without having to pass props manually at every level. It's particularly useful for managing global state or sharing data that's needed by many components.
// Example of using Context API for global state management
import React, { createContext, useState } from 'react';
const UserContext = createContext();
const UserProvider = ({ children }) => {
const [user, setUser] = useState(null);
return (
<UserContext.Provider value={{ user, setUser }}>
{children}
</UserContext.Provider>
);
};
export { UserContext, UserProvider };
State Management Libraries:
For more complex state management needs, you can leverage libraries like Redux or MobX. These libraries offer centralized state management solutions with powerful features such as middleware, time-travel debugging, and immutable state updates.
// Example of Redux store configuration
import { createStore } from 'redux';
import rootReducer from './reducers';
const store = createStore(rootReducer);
React Router:
React Router is a popular routing library for React applications, enabling declarative routing and navigation between different views or pages within a single-page application (SPA).
// Example of React Router configuration
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
const App = () => {
return (
<Router>
<Switch>
<Route exact path="/" component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
</Switch>
</Router>
);
};
By mastering these advanced React.js concepts and techniques, you'll be equipped to build more sophisticated and maintainable React applications. In the following chapters, we'll continue to explore advanced topics and best practices for MERN Stack development, empowering you to build high-quality, scalable web applications.
Chapter 9: RESTful API Development with Express.js
In this chapter, we'll dive deeper into building RESTful APIs using Express.js, exploring advanced routing techniques, middleware usage, input validation, authentication, and error handling. By mastering these concepts, you'll be able to develop robust and secure backend APIs for your MERN Stack applications.
Advanced Routing Techniques:
Express.js offers various routing techniques to handle complex routing scenarios effectively. This includes route parameters, route handlers, route chaining, and route composition.
// Example of route parameters
app.get('/api/users/:userId', (req, res) => {
const userId = req.params.userId;
// Logic to fetch user by ID
});
// Example of route chaining
app.route('/api/posts')
.get((req, res) => {
// Logic to fetch all posts
})
.post((req, res) => {
// Logic to create a new post
});
Middleware Usage for Request Processing:
Middleware functions in Express.js are used to perform tasks such as request parsing, authentication, authorization, logging, error handling, and more. They enhance the functionality of route handlers and can be applied globally or locally to specific routes.
// Example of middleware for request logging
app.use((req, res, next) => {
console.log(`[${new Date().toISOString()}] ${req.method} ${req.url}`);
next();
});
// Example of custom middleware for authentication
const authenticate = (req, res, next) => {
// Logic to verify user authentication
if (authenticated) {
next();
} else {
res.status(401).send('Unauthorized');
}
};
app.get('/api/secure-data', authenticate, (req, res) => {
// Logic to access secure data
});
Input Validation and Sanitization:
Validating and sanitizing user input is essential to prevent security vulnerabilities such as injection attacks, cross-site scripting (XSS), and data manipulation. Express.js offers middleware libraries like express-validator
for input validation and sanitization.
// Example of input validation middleware
const { body, validationResult } = require('express-validator');
app.post('/api/users', [
body('email').isEmail(),
body('password').isLength({ min: 6 })
], (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
// Logic to create a new user
});
Authentication and Authorization:
Implementing authentication and authorization mechanisms is crucial for securing your backend APIs. This involves techniques such as token-based authentication, session management, role-based access control (RBAC), and OAuth integration.
// Example of token-based authentication middleware
const jwt = require('jsonwebtoken');
const authenticateToken = (req, res, next) => {
const token = req.headers.authorization.split(' ')[1];
if (token == null) return res.status(401).send('Unauthorized');
jwt.verify(token, process.env.ACCESS_TOKEN_SECRET, (err, user) => {
if (err) return res.status(403).send('Forbidden');
req.user = user;
next();
});
};
app.get('/api/profile', authenticateToken, (req, res) => {
// Logic to fetch user profile
});
Error Handling and Response Formatting:
Express.js provides middleware for handling errors and formatting responses to provide meaningful feedback to clients. This includes handling various types of errors, setting appropriate HTTP status codes, and sending error messages in a consistent format.
// Example of error handling middleware
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Internal Server Error');
});
By mastering these advanced techniques for RESTful API development with Express.js, you'll be able to build secure, scalable, and feature-rich backend services to power your MERN Stack applications. In the following chapters, we'll continue to explore additional topics and best practices for MERN Stack development, guiding you towards becoming a proficient full-stack developer.
Chapter 10: Real-Time Communication with WebSockets
In this chapter, we'll explore the concept of real-time communication in web applications using WebSockets. Unlike traditional HTTP requests, which are stateless and one-way, WebSockets enable full-duplex communication channels between clients and servers, allowing for bidirectional data flow in real time. We'll learn how to implement WebSocket functionality in both the backend (using Node.js and Express.js) and frontend (using React.js) of our MERN Stack applications.
Understanding WebSockets:
WebSockets provide a persistent connection between a client (such as a web browser) and a server, enabling low-latency, bidirectional communication. Once established, a WebSocket connection remains open, allowing both the client and server to send messages to each other at any time.
Setting Up WebSocket Server with Node.js and Express.js:
We can use the ws
library to implement WebSocket functionality in our Node.js backend. First, install the library using npm:
npm install ws
Next, create a WebSocket server in your Express.js backend:
// backend/server.js
const express = require('express');
const http = require('http');
const WebSocket = require('ws');
const app = express();
const server = http.createServer(app);
const wss = new WebSocket.Server({ server });
wss.on('connection', (ws) => {
console.log('Client connected');
ws.on('message', (message) => {
console.log(`Received message: ${message}`);
// Handle incoming messages from clients
});
ws.send('Welcome to the WebSocket server!');
});
server.listen(3001, () => {
console.log('WebSocket server is running on port 3001');
});
Implementing WebSocket Client in React.js:
In the React.js frontend of our MERN Stack application, we can use the WebSocket
API or libraries like socket.io-client
to establish a WebSocket connection with the server and exchange messages.
// frontend/client/src/components/WebSocketClient.js
import React, { useEffect } from 'react';
const WebSocketClient = () => {
useEffect(() => {
const ws = new WebSocket('ws://localhost:3001');
ws.onopen = () => {
console.log('WebSocket connected');
};
ws.onmessage = (event) => {
console.log(`Received message: ${event.data}`);
// Handle incoming messages from the server
};
return () => {
ws.close();
};
}, []);
return (
<div>
<h2>WebSocket Client</h2>
{/* Add WebSocket client UI components */}
</div>
);
};
export default WebSocketClient;
Real-Time Features and Use Cases:
WebSocket technology opens up a wide range of possibilities for real-time features in web applications, including live chat, real-time collaboration, online gaming, live notifications, stock market updates, and more. By leveraging WebSockets, you can enhance user experience and engagement in your MERN Stack applications.
Scaling and Deployment Considerations:
When deploying MERN Stack applications that utilize WebSockets, it's essential to consider scalability and deployment strategies. Load balancing, horizontal scaling, session affinity, and WebSocket connection management are crucial factors to ensure optimal performance and reliability in production environments.
By incorporating WebSockets into your MERN Stack applications, you can create interactive, real-time experiences that keep users engaged and informed. In the following chapters, we'll continue to explore advanced topics and best practices for MERN Stack development, guiding you towards building high-quality, feature-rich web applications.
Chapter 11: Securing Your MERN Stack Applications
Security is paramount in any web application, and MERN Stack applications are no exception. In this chapter, we'll explore best practices for securing your MERN Stack applications at various levels, including frontend security, backend security, authentication, authorization, data validation, and protection against common security vulnerabilities.
Frontend Security Measures:
-
Cross-Site Scripting (XSS) Prevention: Sanitize user inputs and escape special characters to prevent XSS attacks. Use Content Security Policy (CSP) headers to restrict resource loading and execution.
-
Cross-Site Request Forgery (CSRF) Protection: Implement CSRF tokens in forms and AJAX requests to prevent unauthorized requests initiated by malicious sites.
-
Secure Transmission: Ensure all communication between the client and server is encrypted using HTTPS to prevent eavesdropping and man-in-the-middle attacks.
Backend Security Practices:
-
Input Validation: Validate and sanitize all user inputs on the server-side to prevent injection attacks, such as SQL injection and NoSQL injection.
-
Authentication Mechanisms: Implement strong authentication mechanisms, such as JSON Web Tokens (JWT), OAuth, or session-based authentication, to verify the identity of users and protect sensitive endpoints.
-
Authorization Controls: Enforce access control and authorization checks on every request to ensure that users only have access to resources they're authorized to access.
Authentication and Authorization:
-
JWT Authentication: Use JWT tokens for authenticating users and securing API endpoints. Store user credentials securely and issue JWT tokens upon successful authentication.
-
Role-Based Access Control (RBAC): Implement RBAC to define roles and permissions for users, restricting access to certain resources based on their roles.
-
OAuth Integration: Integrate OAuth providers like Google, Facebook, or GitHub for third-party authentication and authorization, reducing the burden of managing user credentials.
Data Validation and Sanitization:
-
Input Validation: Validate and sanitize all user inputs, including form submissions, query parameters, and request bodies, to prevent injection attacks and data manipulation.
-
Schema Validation: Use schema validation libraries like Joi or Yup to validate request payloads against predefined schemas, ensuring data integrity and consistency.
Protection Against Common Security Vulnerabilities:
-
SQL Injection (SQLi) and NoSQL Injection: Use parameterized queries and ORM libraries to prevent SQL injection attacks in SQL databases. Sanitize inputs and use safe query methods to prevent NoSQL injection in NoSQL databases.
-
Cross-Site Scripting (XSS): Sanitize user inputs and escape special characters to prevent XSS attacks. Implement CSP headers to mitigate the impact of XSS vulnerabilities.
-
Cross-Site Request Forgery (CSRF): Implement CSRF tokens and enforce the SameSite attribute in cookies to prevent CSRF attacks.
By implementing these security measures and best practices, you can significantly reduce the risk of security vulnerabilities and protect your MERN Stack applications and user data from malicious actors. In the next chapter, we'll delve into performance optimization techniques for MERN Stack applications, ensuring fast and responsive user experiences.
Chapter 12: Optimizing Performance in MERN Stack Applications
Performance optimization is crucial for ensuring fast loading times, responsive user interfaces, and efficient use of server resources in MERN Stack applications. In this chapter, we'll explore various techniques and best practices for optimizing the performance of both frontend and backend components of your MERN Stack applications.
Frontend Performance Optimization:
-
Minification and Compression: Minify and compress CSS, JavaScript, and HTML files to reduce file sizes and decrease load times. Utilize tools like UglifyJS, Terser, and Babel for JavaScript minification.
-
Lazy Loading: Implement lazy loading for images, components, and resources that are not immediately visible on the screen. Lazy loading helps reduce initial page load times by only loading content when it's needed.
-
Code Splitting: Split large JavaScript bundles into smaller chunks to improve initial page load performance. Use tools like Webpack's code splitting feature or dynamic imports in React to achieve code splitting.
-
Optimized Images: Optimize images for the web by compressing them without sacrificing quality. Use image optimization tools or libraries like ImageMagick, TinyPNG, or imagemin to reduce image file sizes.
-
Cache Control: Leverage browser caching by setting appropriate cache-control headers for static assets. Use techniques like cache busting and versioning to ensure that updated assets are fetched when necessary.
Backend Performance Optimization:
-
Database Indexing: Create indexes on frequently queried fields in your MongoDB database to improve query performance. Analyze query execution plans and use tools like MongoDB Compass to identify potential indexing opportunities.
-
Query Optimization: Optimize database queries by using efficient query operators, aggregation pipelines, and query hints. Avoid unnecessary data fetching and processing by selecting only the required fields and using pagination for large datasets.
-
Caching: Implement caching mechanisms to store frequently accessed data in memory or a dedicated caching layer (e.g., Redis) to reduce database load and improve response times. Use caching strategies like time-based expiration or cache invalidation to keep cached data up to date.
-
Asynchronous Operations: Utilize asynchronous programming techniques, such as async/await or Promises, to handle I/O-bound operations efficiently. Avoid blocking operations that can degrade server performance, such as synchronous file I/O or CPU-intensive computations.
-
Connection Pooling: Configure connection pooling in your Node.js application to reuse database connections and minimize connection overhead. Use connection pool management libraries like generic-pool or pg-pool for PostgreSQL.
Frontend and Backend Performance Monitoring:
-
Performance Profiling: Use performance profiling tools like Chrome DevTools, Lighthouse, or WebPageTest to identify performance bottlenecks in your frontend code. Analyze metrics such as First Contentful Paint (FCP), Time to Interactive (TTI), and Total Blocking Time (TBT) to optimize rendering and interaction times.
-
Backend Monitoring: Monitor server-side performance metrics, such as CPU usage, memory usage, request throughput, and response times, using monitoring tools like New Relic, Datadog, or Prometheus. Set up alerts for abnormal performance fluctuations to detect and resolve issues proactively.
By implementing these performance optimization techniques and continuously monitoring the performance of your MERN Stack applications, you can deliver fast, responsive, and scalable user experiences to your users. In the next chapter, we'll explore techniques for deploying and scaling MERN Stack applications in production environments, ensuring reliability and availability.
Chapter 13: Deploying and Scaling MERN Stack Applications
Deploying and scaling MERN Stack applications in production environments is essential to ensure reliability, availability, and performance under varying loads. In this chapter, we'll explore deployment strategies, cloud hosting options, containerization, orchestration, and scaling techniques to effectively manage and scale your MERN Stack applications.
Deployment Strategies:
-
Traditional Server Deployment: Deploy your MERN Stack application to a traditional server environment using platforms like AWS EC2, DigitalOcean, or Google Cloud Compute Engine. Manually configure and manage the server infrastructure, including operating system, web server (e.g., Nginx), and database server.
-
Platform-as-a-Service (PaaS): Use PaaS providers like Heroku, AWS Elastic Beanstalk, or Google App Engine to deploy and manage your MERN Stack applications without worrying about server infrastructure management. PaaS platforms offer automated scaling, deployment pipelines, and monitoring capabilities out of the box.
-
Containerization with Docker: Containerize your MERN Stack application components using Docker containers to ensure consistency and portability across different environments. Docker enables packaging applications and their dependencies into lightweight, isolated containers that can be easily deployed and scaled.
-
Container Orchestration with Kubernetes: Orchestrate and manage Docker containers at scale using Kubernetes, an open-source container orchestration platform. Kubernetes provides features like automated deployment, scaling, load balancing, and self-healing to streamline the management of containerized applications in production environments.
Cloud Hosting Options:
-
AWS (Amazon Web Services): Leverage AWS services like Amazon EC2, Amazon RDS (for MongoDB), Amazon S3 (for file storage), and AWS Elastic Beanstalk for deploying and scaling your MERN Stack applications on the cloud. Use AWS CloudFormation or AWS Amplify for infrastructure as code (IaC) and deployment automation.
-
Google Cloud Platform (GCP): Deploy your MERN Stack applications on GCP using services like Google Compute Engine, Google Kubernetes Engine (GKE), Firebase (for hosting and backend services), and Cloud SQL (for MongoDB). Take advantage of GCP's global infrastructure and managed services for scalability and reliability.
-
Microsoft Azure: Utilize Azure services like Azure Virtual Machines, Azure Kubernetes Service (AKS), Azure App Service, and Azure Cosmos DB (for MongoDB) to deploy and scale your MERN Stack applications on Microsoft's cloud platform. Azure offers integrated development and deployment tools for streamlined application lifecycle management.
Scaling Techniques:
-
Vertical Scaling: Increase the compute and memory resources of individual servers to handle increased load. Vertical scaling involves upgrading server hardware or migrating to more powerful instances in the cloud to accommodate growing application demands.
-
Horizontal Scaling: Scale out your MERN Stack application horizontally by adding more instances (nodes) to the server pool. Load balancers distribute incoming traffic across multiple instances, allowing you to handle higher request volumes and improve fault tolerance.
-
Auto Scaling: Implement auto-scaling policies to dynamically adjust the number of application instances based on predefined metrics like CPU utilization, memory usage, or request throughput. Auto-scaling ensures optimal resource utilization and cost efficiency by automatically scaling resources up or down in response to changing demand.
By selecting the appropriate deployment strategy, cloud hosting option, and scaling techniques for your MERN Stack applications, you can ensure seamless deployment, high availability, and efficient resource utilization in production environments. In the final chapter, we'll summarize key takeaways and provide additional resources for further learning and exploration in MERN Stack development.
Chapter 14: Summary and Further Learning
Congratulations on completing this comprehensive guide to MERN Stack development! Throughout this book, we've covered a wide range of topics, including MongoDB, Express.js, React.js, Node.js, real-time communication with WebSockets, security, performance optimization, deployment, and scaling. Let's summarize the key takeaways and provide resources for further learning and exploration in MERN Stack development.
Key Takeaways:
-
MongoDB: MongoDB is a popular NoSQL database that offers flexibility, scalability, and high performance for storing and managing data in MERN Stack applications. It features a flexible document model, powerful querying capabilities, and built-in replication and sharding for horizontal scalability.
-
Express.js: Express.js is a minimalist web application framework for Node.js that simplifies the process of building backend APIs and web servers. It provides a robust set of features for routing, middleware, templating, and error handling, making it ideal for developing RESTful APIs and microservices.
-
React.js: React.js is a declarative and component-based JavaScript library for building user interfaces in MERN Stack applications. It enables developers to create interactive and reusable UI components, manage state efficiently, and implement virtual DOM rendering for optimal performance.
-
Node.js: Node.js is a server-side JavaScript runtime environment that allows developers to build scalable and high-performance web applications. It leverages event-driven, non-blocking I/O to handle concurrent requests efficiently, making it well-suited for building real-time applications, APIs, and microservices.
-
Real-Time Communication: WebSockets enable bidirectional, real-time communication between clients and servers in MERN Stack applications. They provide persistent connections that facilitate instant data exchange, making them ideal for implementing features like live chat, real-time collaboration, and live notifications.
-
Security and Performance Optimization: Security and performance are critical aspects of MERN Stack development. Implement best practices for securing your applications against common vulnerabilities like XSS, CSRF, and injection attacks. Optimize frontend and backend performance through techniques like code minification, lazy loading, caching, and database optimization.
-
Deployment and Scaling: Choose the appropriate deployment strategy and cloud hosting option for your MERN Stack applications based on factors like scalability, reliability, and cost. Implement scaling techniques such as vertical scaling, horizontal scaling, and auto-scaling to ensure your applications can handle varying levels of traffic and workload demands.
Further Learning Resources:
-
MongoDB University: Offers free online courses on MongoDB, including introductory and advanced topics in database design, querying, and administration.
-
Express.js Documentation: Official documentation provides comprehensive guides, tutorials, and API references for learning Express.js development.
-
React.js Documentation: Official documentation contains tutorials, guides, and API references for mastering React.js development, including hooks, context, and concurrent mode.
-
Node.js Documentation: Official documentation offers guides, tutorials, and API references for learning Node.js development, including asynchronous programming, event-driven architecture, and package management.
-
Fullstackopen: A free online course by the University of Helsinki covering full-stack development with React.js, Node.js, Express.js, and MongoDB.
-
Real-Time Web with WebSocket: Book by Simon Holmes provides practical examples and best practices for implementing real-time communication with WebSockets in web applications.
-
Scaling Web Applications: Book by Artur Ejsmont covers strategies and techniques for deploying, scaling, and managing web applications in production environments.
By continuing to explore these resources and applying the knowledge and skills gained from this book, you'll become proficient in MERN Stack development and be well-equipped to build robust, scalable, and feature-rich web applications. Good luck on your MERN Stack journey!
Chapter 15: Conclusion
In this comprehensive guide to MERN Stack development, we've covered everything you need to know to build modern, full-stack web applications using MongoDB, Express.js, React.js, and Node.js. From understanding the fundamentals of each technology to mastering advanced concepts like real-time communication, security, performance optimization, deployment, and scaling, you've gained valuable insights and skills to become a proficient MERN Stack developer.
As you embark on your journey in MERN Stack development, remember to continuously practice, explore new technologies, and stay updated with the latest trends and best practices in web development. Whether you're building personal projects, contributing to open-source initiatives, or working on professional endeavors, the knowledge and experience you've gained from this guide will serve as a solid foundation for success.
Always strive to build user-centric, scalable, and maintainable web applications that deliver exceptional user experiences and add value to people's lives. Embrace challenges, learn from failures, and celebrate successes as you continue to grow and evolve as a developer.
Thank you for joining us on this journey through the MERN Stack ecosystem. We hope this guide has provided you with valuable insights, inspiration, and practical knowledge to thrive in the dynamic world of web development. Remember, the possibilities are endless when you have the skills and creativity to bring your ideas to life.
Wishing you all the best on your MERN Stack adventures! Happy coding!